Organizational and Gantt Hierarchy Styling
Organizational chart and Gantt chart hierarchy and dependency customization.
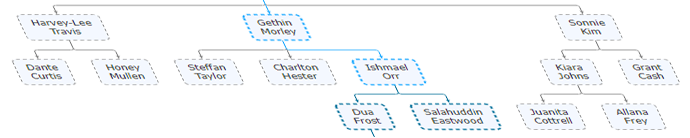
Introduction
Customization and control over org relationship paths and Gantt dependencies is provided through hierarchy paths, similar to breadcrumbs. These paths are used to customize the connector lines and point annotations between points in the hierarchy. For example, you can highlight the path from the current hovered point to the top point in the hierarchy or change the styling of the path and points when clicking a given point.
Style Connector Lines
You can define styling of connector lines for each point individually, and each parent (for points with multiple parents).
All connector lines for points in a series can be styled with Series.defaultPoint.connectorLine properties. Point.connectorLine allows setting parent connector line styling per point, including connectorLine_radius to control the corner rounding. Line styling can also be set for individual parent connectors when multiple parents are defined as detailed in the following Specifying Parent Points section.
Specifying Parent Points
Parent points are specified by point ID.
Within the context of point object such as:
var chartOptions = {
series: [
{
points: [
{ id: "id", name: "Node id" },
{ id: "id2", name: "Node id2" },
{ id: "id3", name: "Node id3" },
{ id: "id4", name: "Node id4", parent: "id" }
]
}
]
};
The simple way to specify parents for point id4 above through the point.parent property is with:
parent: 'id', or 'id,id2'You can also define the point connector with ID and line style through the parent property.
parent: {id:'id', line: {color: 'blue'}} or {id: 'id,id2', line: {color: 'blue'}}Additionally, you can specify an array of point connectors. This example defines 3 parent connectors, one with a blue line and two with a green line style.
var chartOptions = {
series: [
{
points: [
{ id: "id", name: "Node id" },
{ id: "id2", name: "Node id2" },
{
id: "id3",
name: "Node id4",
parent: [{ id: "id", line: { color: "blue" } }, { id: "id2,id3", line: { color: "green" } }]
}
]
}
]
};
Highlight a connector path
To highlight a path between two points or from a point all the way up or down the hierarchy, use the chart.connectors() function.
chart.connectors(pathSelector [, style] [, updateOptions])Path Selectors
The syntax for path selectors is
['pointID', (PointID2 or Direction)]The direction can be one of the following keywords: up, down, (both or all)
This example makes all connector lines from pointID to the top of the hierarchy red:
chart.connectors(["pointID", "up"], { color: "red" });
More examples of path selectors:
// All branches from pointID down the hierarchy.
(["pointID", "down"],
// All branches from pointID up and down the hierarchy.
["pointID", "both"],
// All branches between pointA and pointB
["pointA", "pointB"],
// A specific branch of points.
["pointA", "pointB", "pointC"],
// Multiple branches and selectors.
[["pointA", "pointC"], ["pointC", "up"]])
This function returns an array of paths where each path is an array of point IDs that are connected. If there are multiple paths in your selection, they will all be returned in the array.
The following are some examples using the chart.connector() function. All these examples return arrays of paths.
/* Style the specified connector lines red.*/
chart.connectors(["pointID", "up"], { color: "red" });
/* Do nothing except return the paths involved.*/
chart.connectors(["pointID", "up"], {});
/* Reset the specified connector styles to their original state.*/
chart.connectors(["pointID", "up"]);
/* Reset all connector lines to their original state.*/
chart.connectors();
Individual paths (arrays of points) can be used as path selectors to style individual paths with the Chart.connectors() function as well.
When connectors are styled this way, multiple calls to the Chart.connectors() function apply the styling updates to the prior styles. If you wish to reset styles to the original styling, all styles set in this manner can be reset by calling Chart.connectors() without any arguments.To style a new path only, call Chart.connectors() without any arguments first to reset all styles, then call it again with a path and style to apply it only to the specific path.
When using the Chart.connectors() function to get a list of paths from a pathSelector and to avoid resetting other styles you have applied, use an empty object for line styling. For example:
var paths = chart.connectors(["pointID", "up"], {});
Without this empty object, the selected paths are reset to their original states.
Highlight points along the path
To style the points along these paths, you can use the same path selector with the Chart.series().points() function to select them.
chart.series().points([pointID, "up"]);
It is then recommended that you use the point selected or muted states to style these points rather than any specific styling options such as color properties, because the selected and muted states apply very efficiently and can be used with point hover events maintaining the highest performance. For example, to highlight paths up the tree when hovering points, use:
chart
.series()
.points([pointID, "up"])
.options({ selected: true });
In the case of Gantt charts, these paths can traverse multiple series and will still work without issues.
Important Notes
- Use point states to modify point styling with mouse hover events to optimize performance.
- When using series.pointSelection=false to apply a style manually, set point_state_selected_enabled:true to ensure the style is created internally.
- When using the mute state for styling, make sure to have a point mouseOut event handler that returns false to prevent automatic mute state changes.
- Styling lines with chart.connectors() supports animation when you specify updateOptions (3rd argument), but not when resetting styles.
Some examples
Directional Hierarchy Highlighting Organizational Chart Sample Highlight organizational hierarchy paths in different directions and style states.
Organizational Chart Path Highlighting and Selection Sample Select and highlight a path from a point up the tree in an organizational chart with custom styles, colors and images using point click, select and mute states.
Point Selection Modes Organizational Chart Sample Try different point selection modes with organizational chart hierarchy highlighting.
Organizational Chart Breadcrumbs Navigation Sample Organizational chart breadcrumbs.
Group Highlight Organizational Chart Sample Customized organizational chart point coloring by role including point and legend highlight on hover.
Critical Path Gantt Chart Sample Toggle visibility of a critical dependency path highlighting.
Manual Tree Traversal
Point objects have Point.getParents() and Point.getChildren() functions that return collections of points. This lets you traverse the hierarchy programmatically to manually analyze the tree data if necessary.